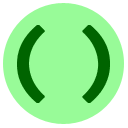
Coding Exercise: Binary Search
Given the length of the sequence
n and the sequence itself (
n integer numbers) and an integer number
m. The given sequence is sorted in increasing order.
Write a program to test if the number
m is among the elements of the sequence. If so, output its index, if not - output 'No'. If number m is met in the sequence several times, output the smallest index.
For example, if
n = 7, the given sequence is 1 3 5 9 12 14 15 and
m = 14, then the output should be 6 (because 14 is the 6'th member of the given sequence).
Solve the problem by using the binary search. You can read more about it:
Binary search You need to create an account and log in to ask a question.